Here is an example of connecting the SQM-LU (or SQM-LU-DL) to an Arduino compatible single board computer.
The Arduino compatible computer I used was the
Teensy 3.2 which is availabke for about $19.80USD.
A USB host interface is required that the SQM-LU can be plugged into. I used the
USB Host Shield V2.0 mini for about $4.85USD available from here:
The USB Host shield 2.0 is documented here:
https://www.circuitsathome.com/category/mcu/arduino/usb-shield/The software library is located here:
https://github.com/felis/USB_Host_Shield_2.0Here is the schematic (
PDF):
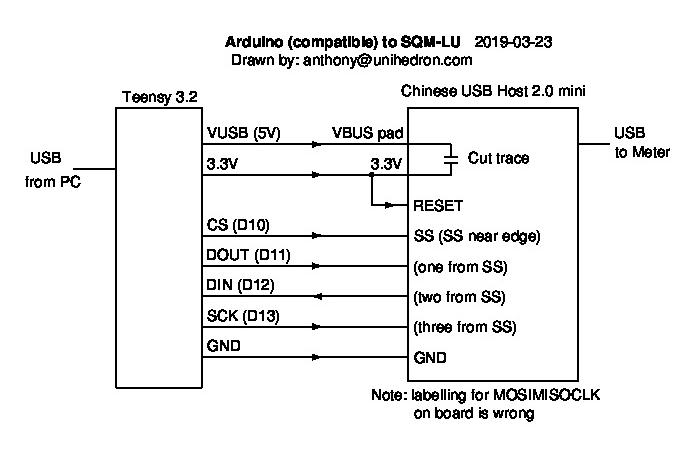
Here is the wiring diagram:
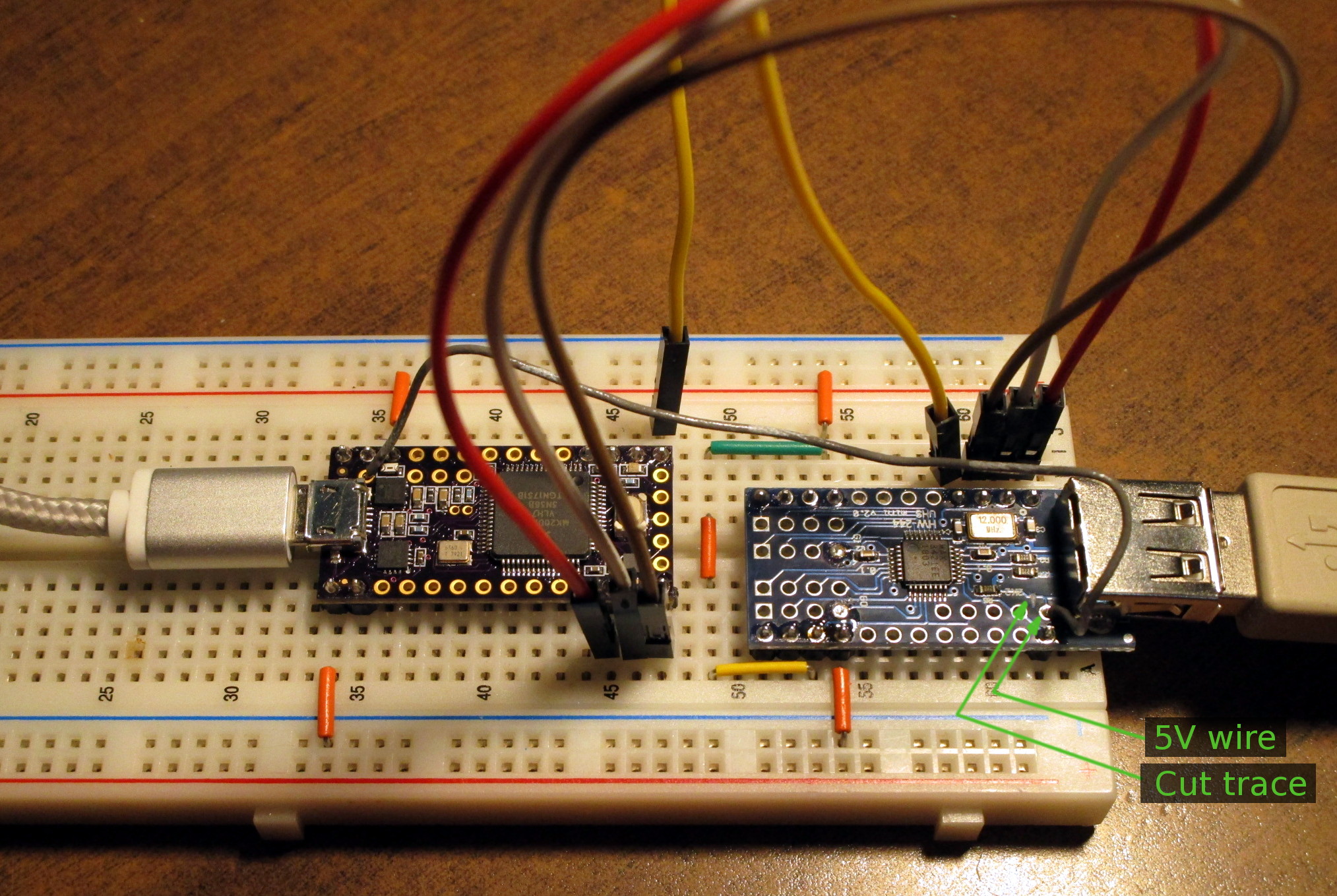
This is the code that I used:
#include <cdcftdi.h>
#include <usbhub.h>
#include "pgmstrings.h"
// Satisfy the IDE, which needs to see the include statment in the ino too.
#ifdef dobogusinclude
#include <spi4teensy3.h>
#endif
#include <SPI.h>
class FTDIAsync : public FTDIAsyncOper
{
public:
uint8_t OnInit(FTDI *pftdi);
};
uint8_t FTDIAsync::OnInit(FTDI *pftdi)
{
uint8_t rcode = 0;
rcode = pftdi->SetBaudRate(115200);
if (rcode)
{
ErrorMessage<uint8_t>(PSTR("SetBaudRate"), rcode);
return rcode;
}
rcode = pftdi->SetFlowControl(FTDI_SIO_DISABLE_FLOW_CTRL);
if (rcode)
ErrorMessage<uint8_t>(PSTR("SetFlowControl"), rcode);
return rcode;
}
USB Usb;
//USBHub Hub(&Usb);
FTDIAsync FtdiAsync;
FTDI Ftdi(&Usb, &FtdiAsync);
void setup() {
Serial.begin( 115200 );
#if !defined(__MIPSEL__)
while (!Serial); // Wait for serial port to connect - used on Leonardo, Teensy and other boards with built-in USB CDC serial connection
#endif
Serial.println("USB Host starting up...");
if (Usb.Init() == -1)
Serial.println("OSC did not start.");
}
void loop()
{
Usb.Task();
if( Usb.getUsbTaskState() == USB_STATE_RUNNING ) {
uint8_t rcode;
char strbuf[] = "rx\r";
rcode = Ftdi.SndData(strlen(strbuf), (uint8_t*)strbuf);
if (rcode){
ErrorMessage<uint8_t>(PSTR("SndData"), rcode);
}
delay(1000); //Wait a while for SQM to respond.
uint8_t buf[64];
for (uint8_t i=0; i<64; i++)
buf[i] = 0;
uint16_t rcvd = 64;
rcode = Ftdi.RcvData(&rcvd, buf);
if (rcode && rcode != hrNAK)
Serial.print("A");
ErrorMessage<uint8_t>(PSTR("Ret"), rcode);
if (rcvd > 2)
Serial.print((char*)(buf+2));
delay(10);
}
}
This is the output on the Arduino serial monitor:
USB Host starting up...
r, 09.37m,0000015912Hz,0000000000c,0000000.000s, 020.6C,D
r, 09.37m,0000015909Hz,0000000000c,0000000.000s, 020.6C,D
r, 09.37m,0000015909Hz,0000000000c,0000000.000s, 020.6C,D
r, 09.37m,0000015909Hz,0000000000c,0000000.000s, 020.6C,D
Some interesting troubleshooting notes are here:
https://www.arduino.cn/thread-81435-1-1.html